I went to Tokyo Disney for 2 days, which includes the Tokyo Disney Sea on day one and Tokyo Island on day 2. I played a total of 15 attractions – 7 at DisneySea and 8 at the Disneyland .
For me, Disney is like a recharge station for my spirit, it fills you with imaginations and brightness to your heart. I hope you feel the same!
If you arrived to Tokyo via Shinkansen, you can take the Keiyo train line toward the Maihama station.
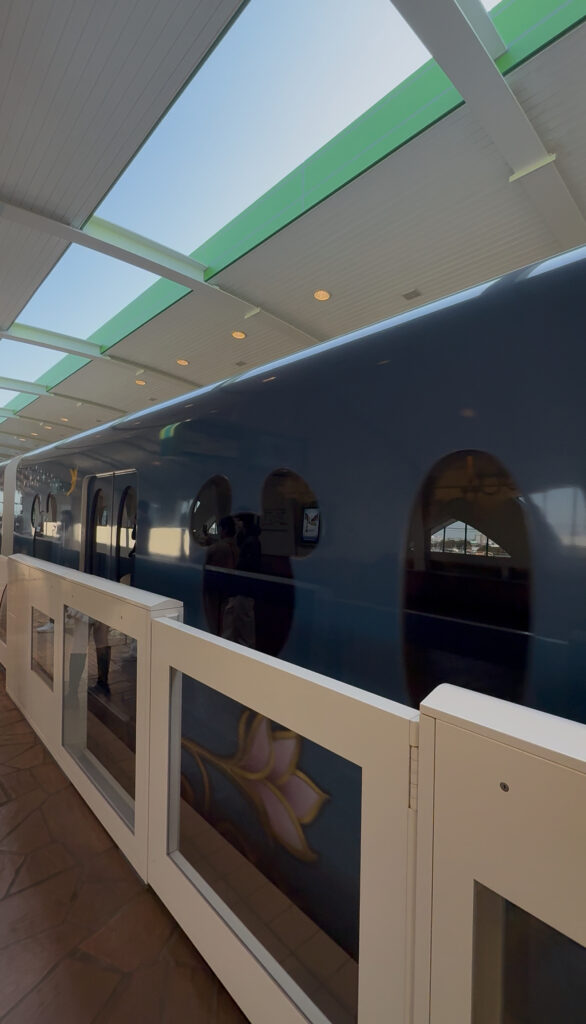
The Disney Resort Line has 4 stations.
When you get off from the JR Keiyo line Maihama station (京葉線 舞浜駅), you are at the Resort Gateway Station.
The train goes in a circle, from Resort Gateway Station (JR Train line) to Tokyo Disneyland Staton, to Bayside Station (hotels), and to the Tokyo DisneySea Station (entrance to Disney Sea).
You can use Suica or Icoca for the train, however, if you are staying for more than a day, you can purchase a 2-day pass:

A 2-day pass allows you to take trains of the Tokyo Disney Resort line at any time.
You can also get a character image there.
There is a key: different ticketing machines issue a different character design. Please pay attention to the image shown on machine screen before making the purchase.
You should 1000% download and use the Disney app, since you will be able to get Standby pass and Disney Premier Access pass to access attractions and shows quickly, and to use mobile orders at restaurants and skip the line (believe me the lines are sometimes long).
I was able to play more than 10 attractions within 2 days by using the Disney Premier Access (DPA), which is a few taps away in the app.
Here is what I played at the park:
Day one (7 attractions) at DisneySea
Arrived at 1PM at Tokyo Disney Sea:
- 20,000 Leagues Under the Sea
Recommendation level: ⭐️⭐️⭐️🌟 Cool
You will go into a submarine and it will go under water to explore.
I did not have to purchase a DPA and the wait is only 10 minutes.
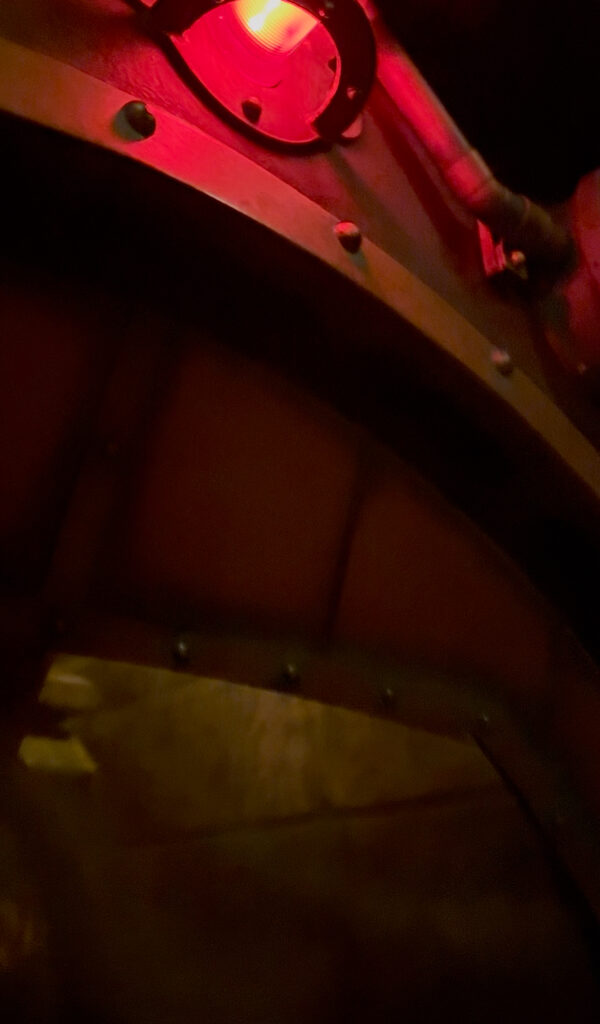
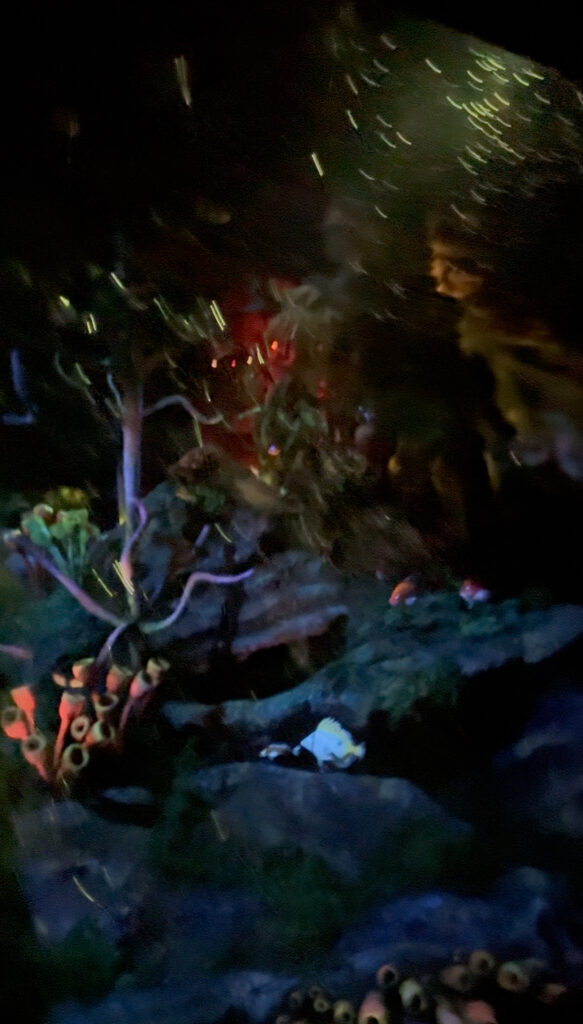
- Journey to the Center of the Earth (DPA)
Recommendation level: ⭐️⭐️⭐️⭐️🌟 MUST
This is super exciting! You will take an excavator and explore within a volcano. At the end of the ride, it will go on roller coaster mode!
Here is the official Disney video:
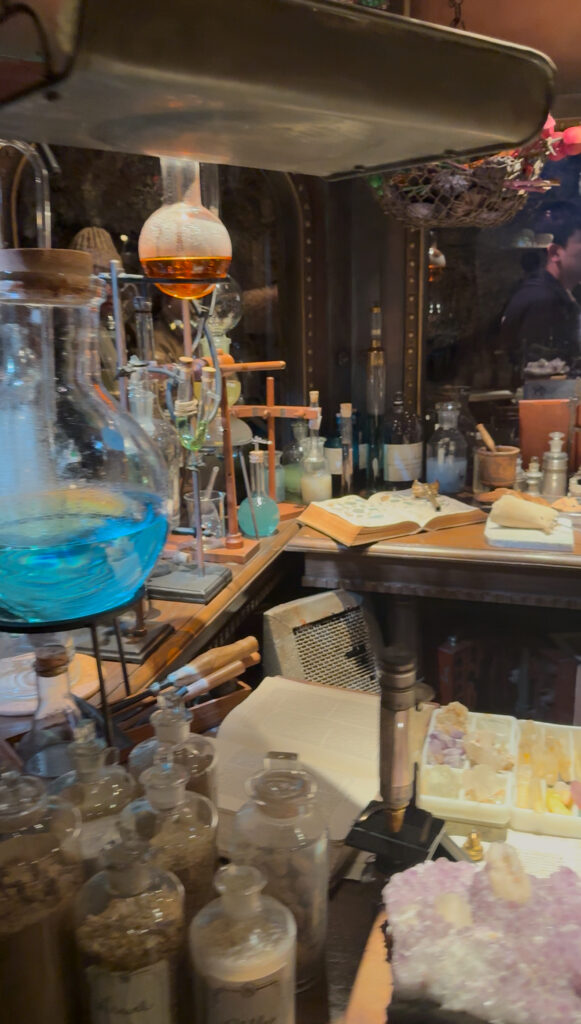
You also get to see captain Nemo’s lab before getting on the ride.
The wait for this is too long! But I purchased the Disney Premier Access within the app and got on the ride within less than 10 minutes.
Here is how to purchase it, it is around $10:
- Register your ticket ahead of time in the Tokyo Disney Resort app
- Use the QR code within the app to enter the park
- Make sure the app has GPS access
- Tap the stars icon at the bottom drawer menu
- Tap Disney Premier Access and continue from there
- Note that after purchasing one of the DPA, you can only purchase the next one either after you complete the current one, or after an hour.
Then, after this ride, I went for the relaxing route of taking a boat in Venice:
- Venetian Gondolas
You can teleport to Venice quickly and enjoy the boat ride. Don’t forget to say Ciao when you pass another boat!
- Checked out the US Steamship
There is a large ship with multiple decks. You can board it and feel free to check it up:

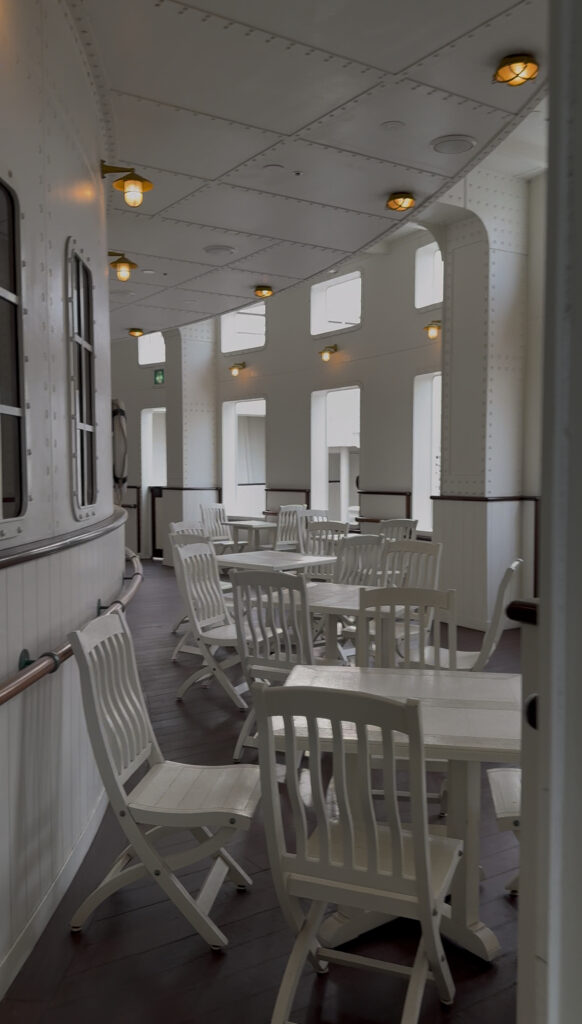
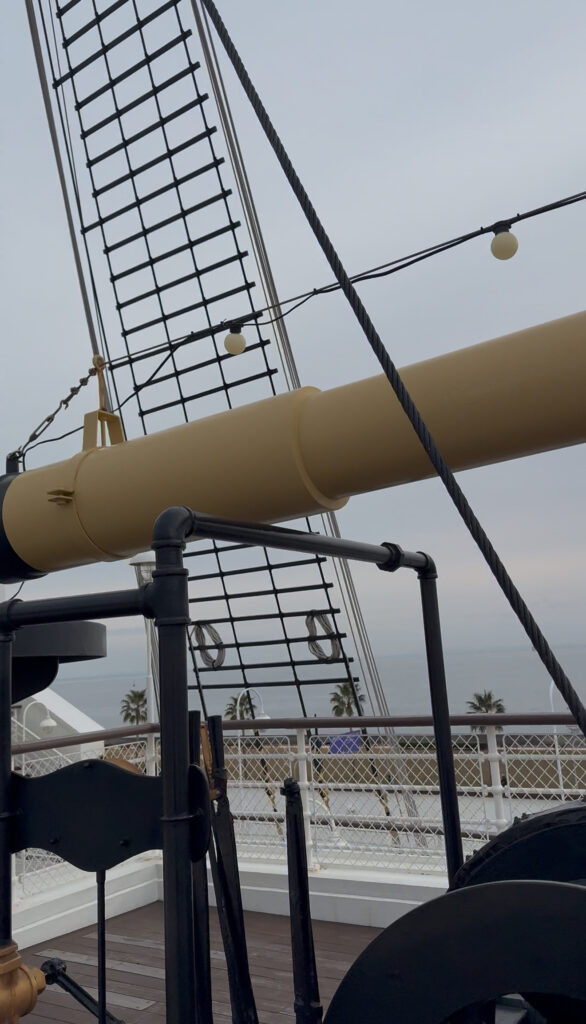


Pro tip: the ship is much more beautiful at night! Don’t forget to take a picture!
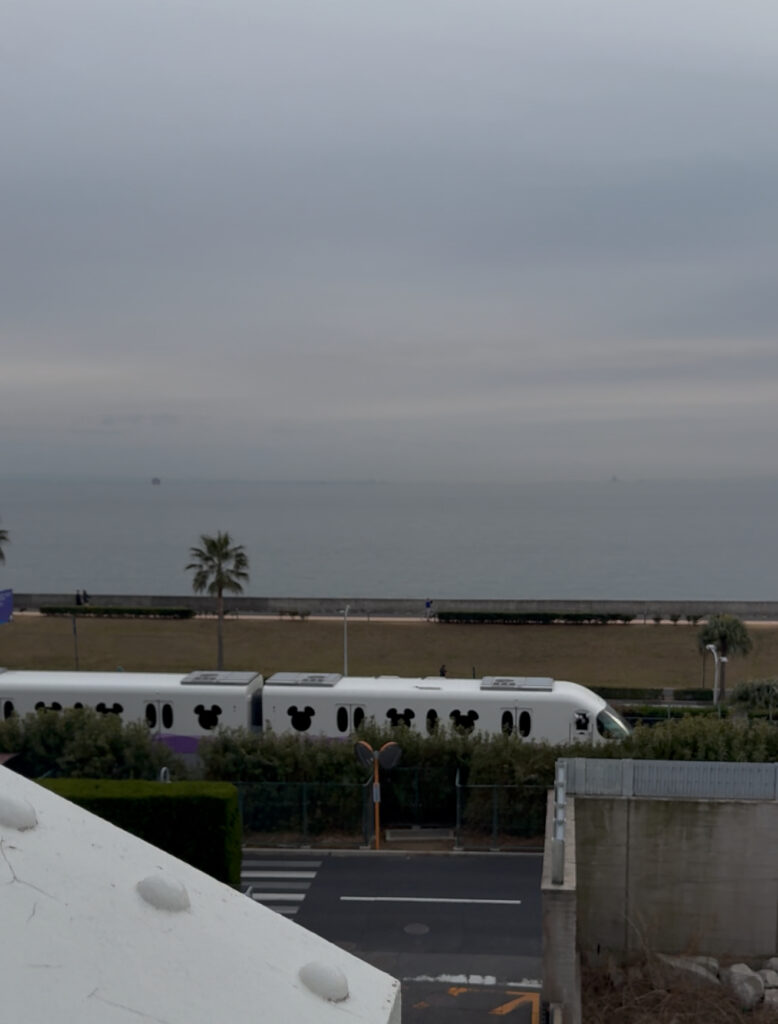
You can see the Disney Resort Line train from on the deck of the boat
- Mickey Mouse greetings
Then, I went to greet Mickey Mouse who was being a “tomb raider” in the Lost River Delta region.



You can give your phone to a crew and they will help you take a picture with Mickey Mouse! Don’t forget to high-five and smile!
- The new area! Fantasy Springs!
Fantasy Springs is a new area opened! There is a beautiful lantern ride (but I did not get the chance to try it 😭 I will try it next time)
- Peter Pan’s Never Land Adventure
I did use the DPA to access the Peter Pan’s Never Land Adventure! It is amazing! The animation and the effects are beyond my imagination. You feel like you are flying with the characters in the infinite sky!
Recommendation level: ⭐️⭐️⭐️⭐️🌟 MUST
Day one was very very exciting! DisneySea is far beyond my imagination! DisneySea feels like a very new park (and indeed it is, with the new Fantasy Springs section)! It has a great layout, and for me, I went from the entrance on the south and played by going all the way North. Until I reach Fantasy Springs (which is on the north side, near the Bayside Disney Resort line station).
The cast members are very very friendly! They are so nice that I remember some of their names and submitted a positive feedback to the DisneySea.
Day Two. Disneyland (8 attractions)
- Big Thunder Mountain
Recommendation level: ⭐️⭐️⭐️🌟 Try it out! The wait is not too long! And it is very exciting, with a photo taken when you slide down to the water.
This is a very exciting small roller coaster, which starts with a trip similar to the This is a Small world, but in the close of the ride, you will go down a large slide into the water! It also takes a picture for you!
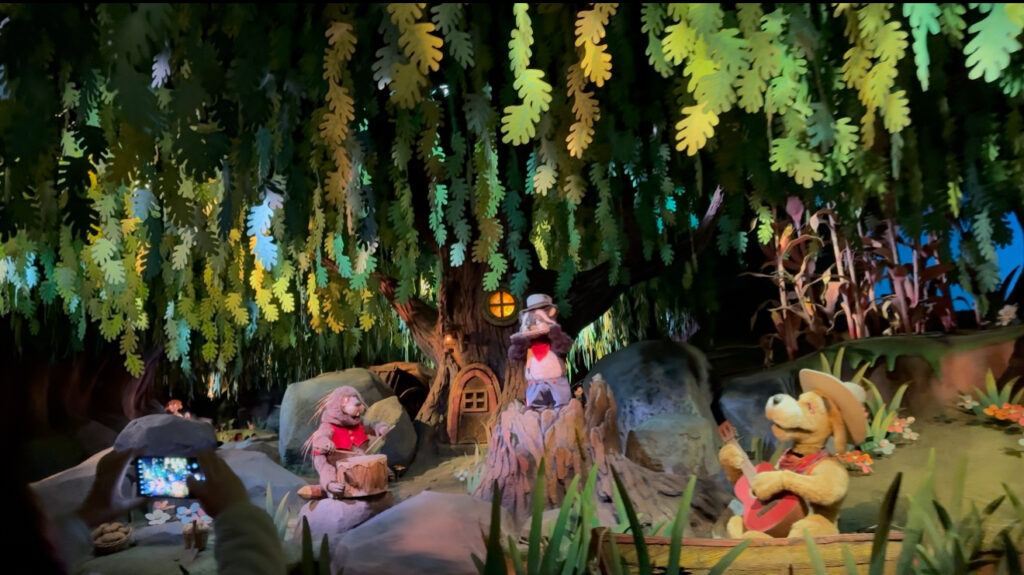



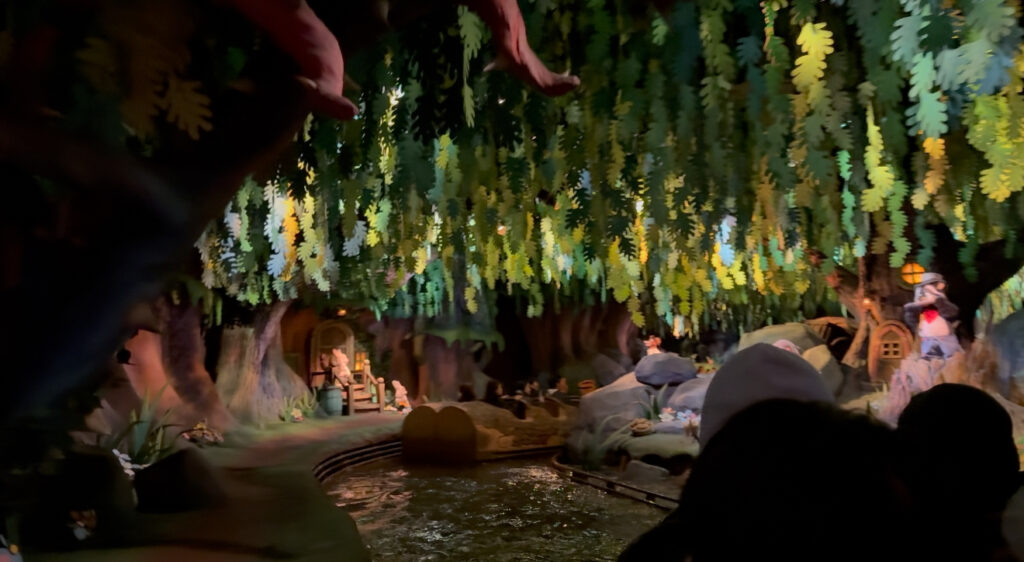
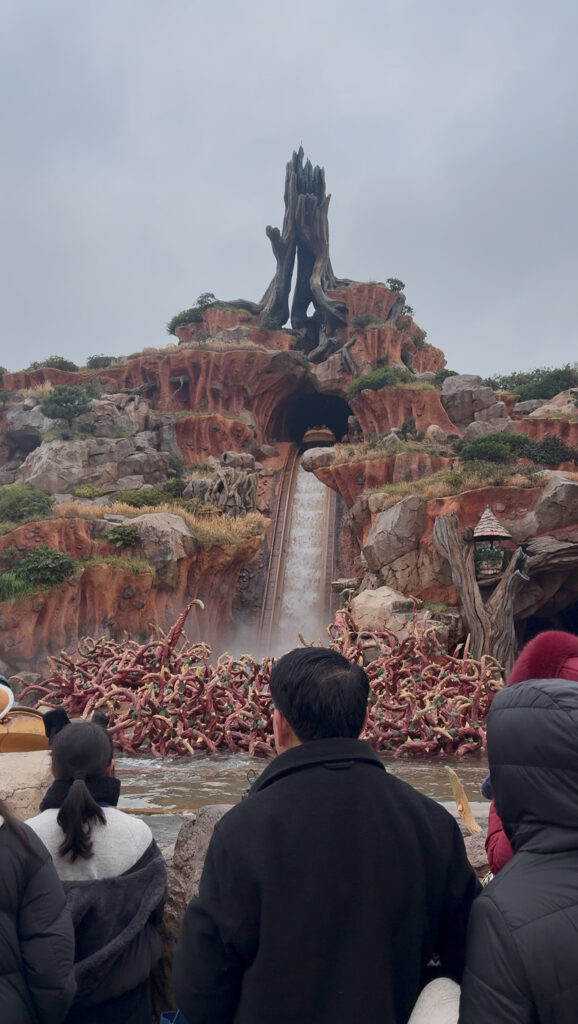
Did you see the boat on top of the slide in the last picture! Yes! That is the most thrilling & exciting part of this ride!
Also, I only had to wait for about 10 minutes, and did not use a DPA here.
- Enchanted Tale of Beauty and the Beast
Recommendation level: ⭐️⭐️⭐️⭐️🌟 MUST
This ride has a super long line of waiting at standby, about 2 hours wait. So I purchased the DPA and got in within 10 minutes.
Before you take on the ride, you will walk into the mansion of the beast and it looks just like in the movie!
This attraction is so well designed, your seat will take you within the rooms of the Beauty and the Beast mansion, take you through the story, and you will join the dance party with the beauty and the beast! It is very high tech and creative!





- The Happy Ride with Baymax
Recommendation level: ⭐️⭐️⭐️⭐️🌟 MUST
This ride is so delight! The music is a hit! And it looks relaxing, but it gets exciting sometimes, with the car moving around quickly!
The wait is super long, around an hour, but a DPA gets me in within 5 minutes.
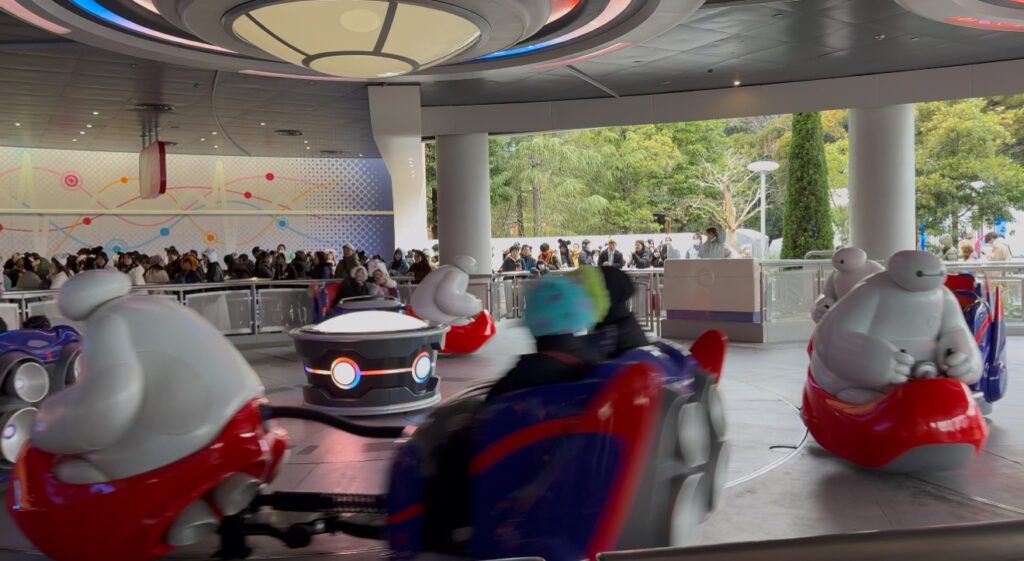
- Club Mouse Beat
For this one, you can try the “Standby Pass” option, since everyone has an assigned seat and there is no wait as like other attractions. However, Standby pass is like a lottery. I did not get the Standby pass, so I used DPA. But you should always try Standby pass first if it is available.

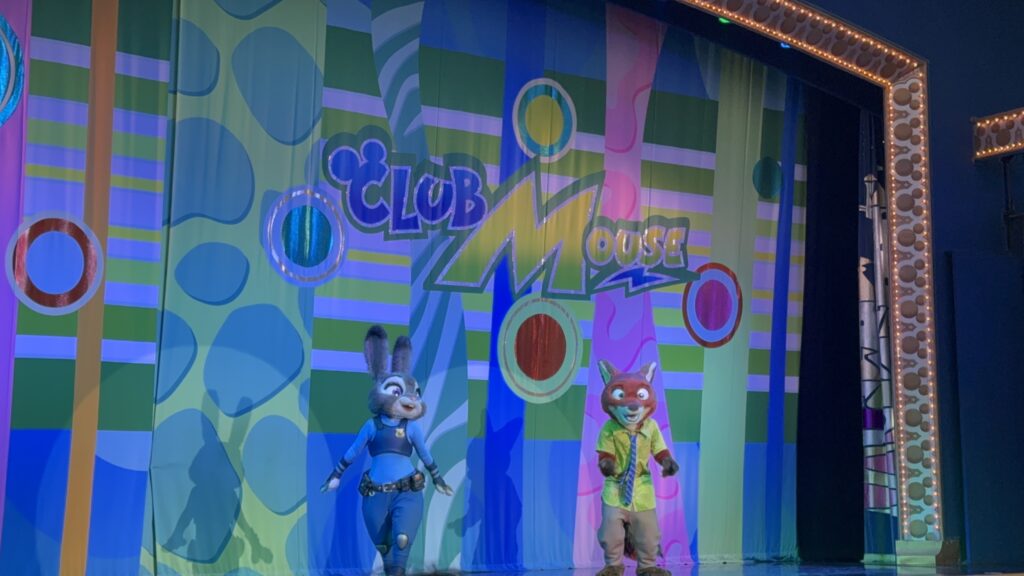
- Parades
There are many parades within the park. You can check it within the Disney Resort app by tapping on the list icon at the bottom right corner (besides the filter funnel) and see a list of shows.

(I love the purple cat!)
- Mickey’s Magical Music World
Recommendation level: ⭐️⭐️⭐️⭐️🌟 MUST
This is very very touching! You see many of your favorite Disney characters show up, including Judy and Nick from Zootopia, of course Mickey Donald, but also Frozen, the Caribbean’s, and many many more! It takes your memory back in time. And the music and the show are very beautiful.

For this show, you need to reserve a seat via Standby pass or purchase a DPA, similar to other shows.
- Little World
This is a very classic attraction! But there are now Marvel elements like Groot!
- Reach for the Stars fireworks show
Recommendation level: ⭐️⭐️⭐️⭐️🌟 MUST
If you need a mental recharge, this is it. I basically cried, looking at all the childhood Disney characters, and listening to the Reach for the Stars (you’ll find further than you know, if you can dream it, you can do it). It is so inspiring!