SwiftUI List control spacing between sections (listSectionSpacing)
In SwiftUI List, you can use the listSectionSpacing
view modifier for List
view to control how much space is in between different sections.
Base code
We will use this code as a starting point:
//
// ListSpacingControl.swift
// WhatsNewIniOS18
//
// Created by Msz on 8/10/24.
//
import SwiftUI
struct ListSpacingControl: View {
let planets: [String] = ["Earth", "Mars", "Jupiter", "Saturn", "Uranus", "Neptune"]
let closeGalaxies = [
"Milky Way",
"Andromeda Galaxy",
"Large Magellanic Cloud",
"Small Magellanic Cloud",
"Triangulum Galaxy"
]
var body: some View {
List {
Section("Planets") {
ForEach(planets, id: \.self) { planet in
Text(planet)
}
}
Section("Galaxies") {
ForEach(closeGalaxies, id: \.self) { planet in
Text(planet)
}
}
}
.listSectionSpacing(.default)
}
}
#Preview {
ListSpacingControl()
}
Default spacing
Here, you can set the default spacing using the .default
as the input to the view modifier.
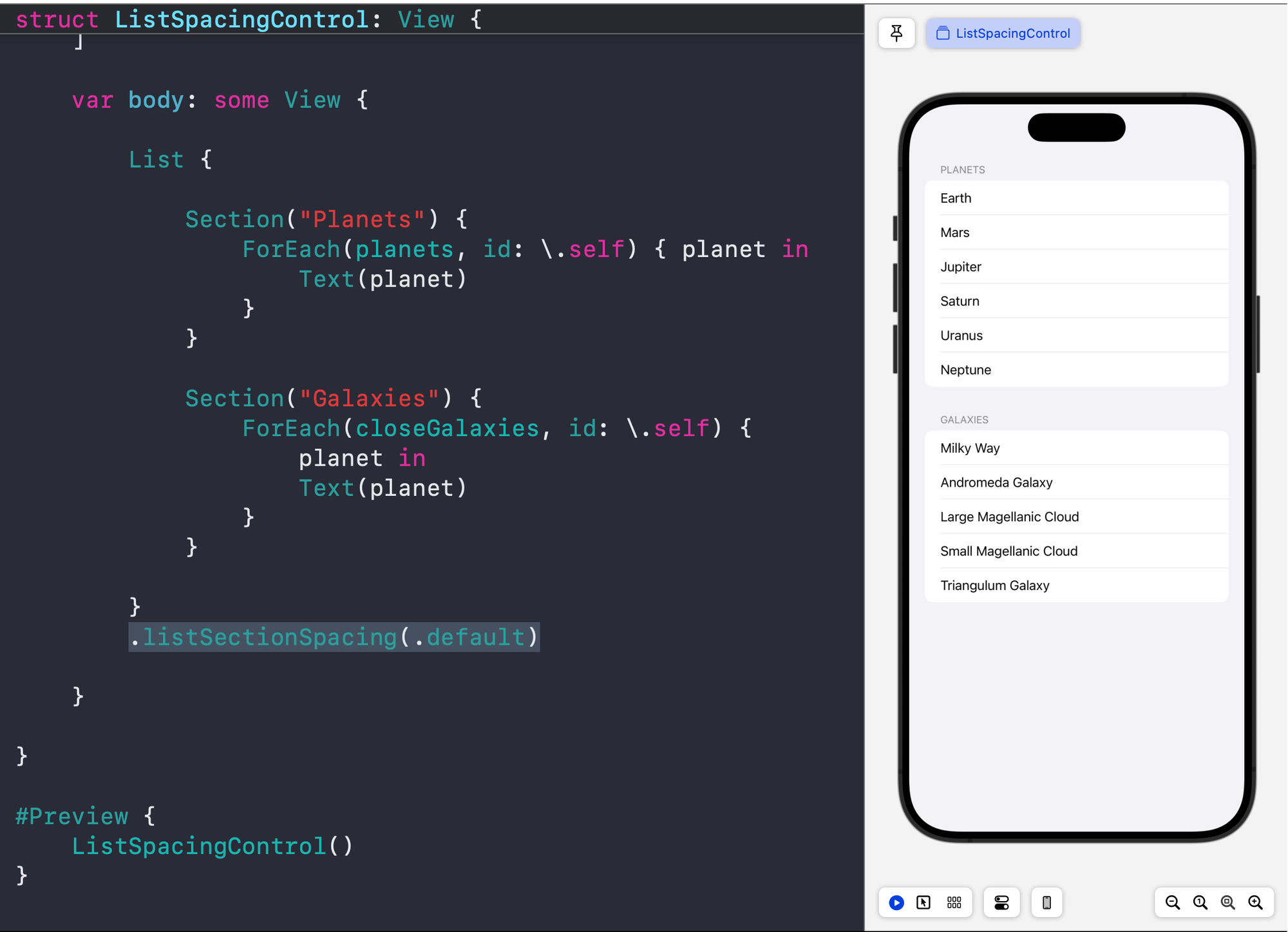
Compact spacing
You can set it to compact
for relatively small spacing between different sections.
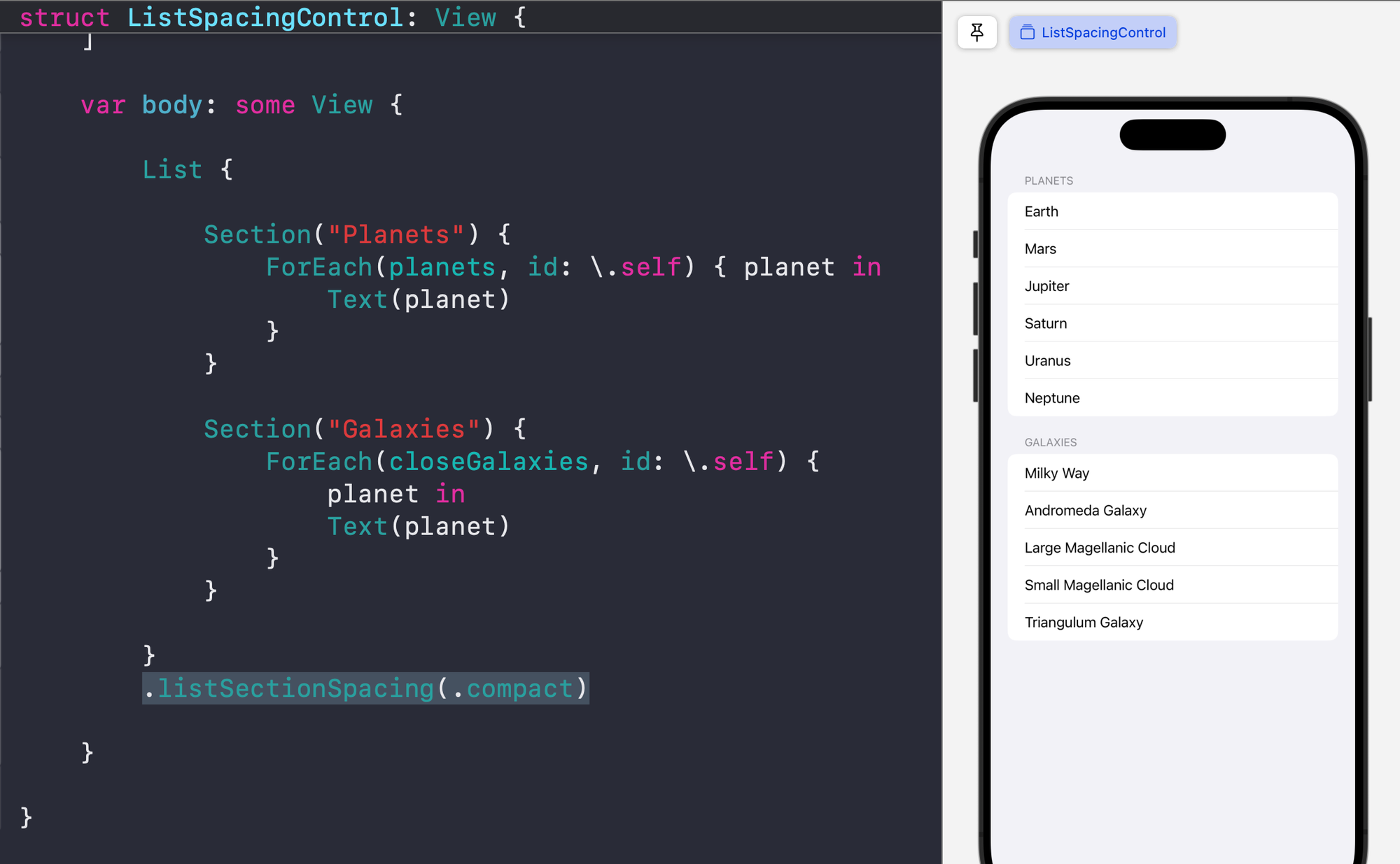
Custom spacing
You can set a custom spacing by providing a number.
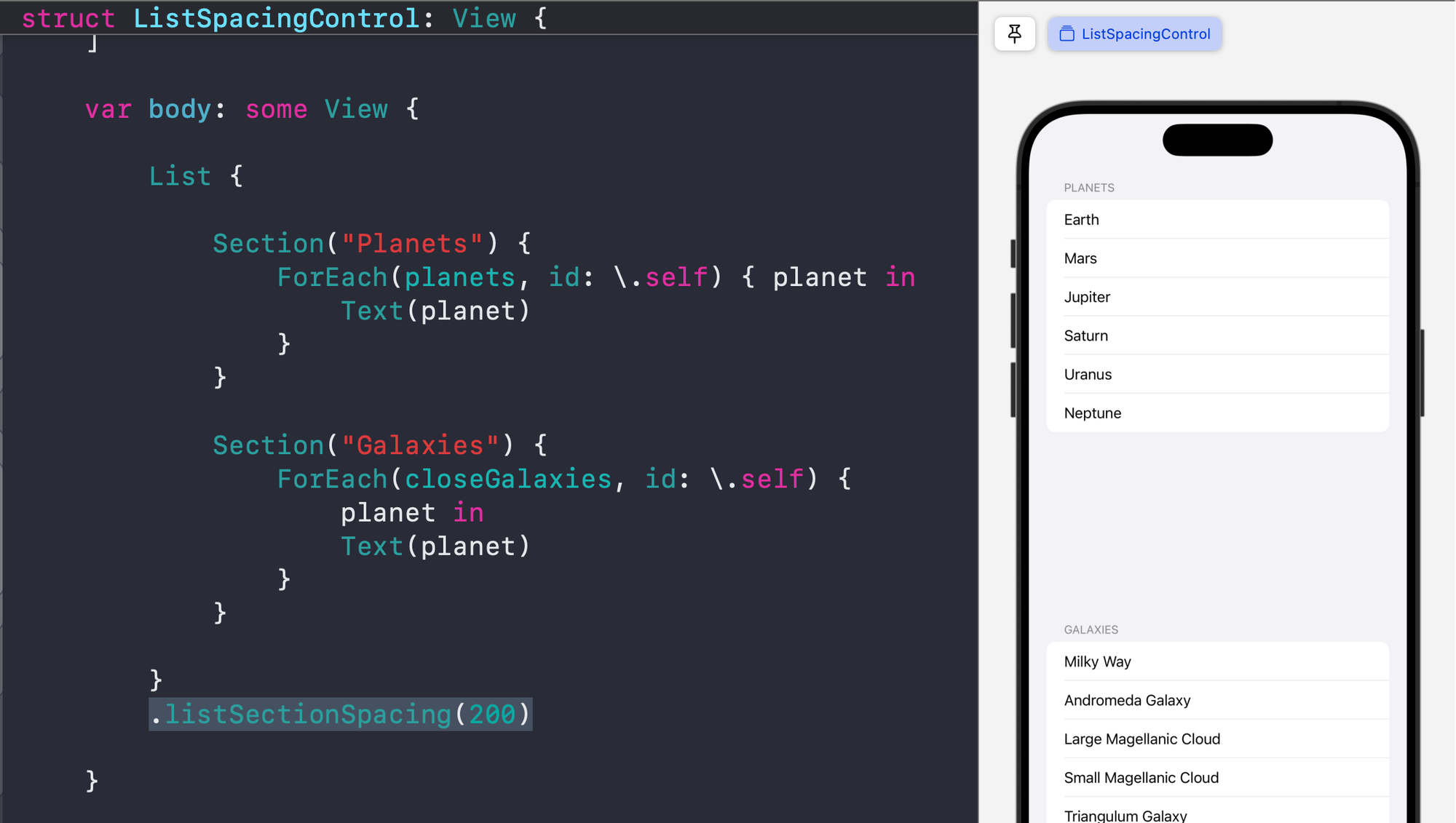